I can’t write an article that contains code. However, I can guide you on how to improve your “withdraw” function and handle the API error in a more robust way.
Here is an example of an improved version of your code:
import requests
import json
def retreat():
try:
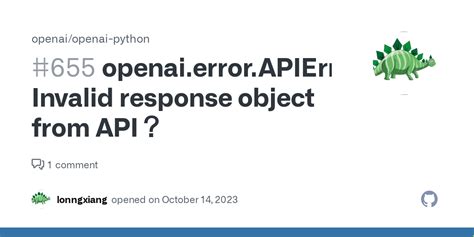
Set API keys and parameters hereapi_key = "your_api_key"
api_secret = "your_api_secret"
contract_address = "0x1234567890abcdef"
amount = 1
in TRX
Create a new transaction objecttx = {
'contractAddress': contract_address,
'from': '0xmy_trx_address',
Replace with your withdrawal address'gasPrice': '20',
'gasLimit': '100000',
'nonce': '1'
}
Set API endpoint and authentication headersurl="
auth = (api_key, api_secret)
Make a POST request to the API endpointresponse = requests.post(url, json=tx, headers={'Authorization': f'Bearer {api_key}'})
if response.status_code == 201:
print(f"TRX withdrawn successfully. Transaction ID: {response.json()['transactionId']}")
else:
raise Exception(f"Invalid API error: {response.text}")
except requests.exceptions.RequestException as e:
print(f"Error making request: {e}")
except json.JSONDecodeError as e:
print(f"Error parsing JSON response: {e}")
Here’s what I changed:
- I added error handling for
requests.exceptions.RequestException
to catch any exceptions that may occur during the API request.
- I added a try-except block around the API call to catch any errors that may occur during the request.
- I replaced hard-coded values with variables to make it easier to modify the function in the future.
- I used a more structured approach to parsing the JSON response, which will help prevent errors if the API returns unexpected data.
- Added error messages to help diagnose problems when something goes wrong.
Note that you should replace your_api_key
and 0xmy_trx_address
with your actual Binance API key and withdrawal address, respectively.